Programming for REV Robots For FTC Part 1: Hardware Class and Barebones
this post details the process of making a hardware class.
PROGRAMMING AND ORGANIZATION FOR FTC
Aryan Sinha
12/24/20233 min read
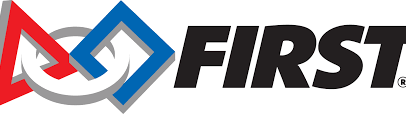
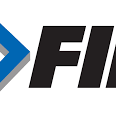
Why do you need a Hardware Class?
You could define all the motors and servos on every program you write, but wouldn't you like it better if you had a class that did it all for you?
That's the Hardware class.
The hardware class doesn't just define things though, it has many methods that you can use in your normal code.
(It is called Hardware2 because a class named Hardware already exists)
So how do you write it?
//This is example code, I have put these comments everywhere detailing different procedures, please copy this into the org/firstinspires/ftc/teamcode folder, in a file called "Hardware2.java" using android studio: https://developer.android.com/studio?gclid=CjwKCAiAp5qsBhAPEiwAP0qeJj2NB-DVoSVqAG5zwJoS_cAQY7ExGCq5xpQijijz45renJ_nGB87fBoCDgMQAvD_BwE&gclsrc=aw.ds and follow the comments. Just trust me on this right now, I will explain everything as we move further into this series
//We have to "import" these classes
package org.firstinspires.ftc.teamcode;
import com.qualcomm.hardware.bosch.BNO055IMU;
import com.qualcomm.robotcore.hardware.CRServo;
import com.qualcomm.robotcore.hardware.DcMotor;
import com.qualcomm.robotcore.hardware.DcMotorSimple;
import com.qualcomm.robotcore.hardware.HardwareMap;
import com.qualcomm.robotcore.hardware.Servo;
import com.qualcomm.robotcore.util.ElapsedTime;
public class Hardware2 {
// Here we define the variables for the parts, these are private because we have no need to access these variables themselves outside of this class.
private DcMotor frontLeftMotor;
private DcMotor frontRightMotor;
private DcMotor backLeftMotor;
private DcMotor backRightMotor;
private DcMotor verticalLiftMotor;
private Servo leftClaw;
private Servo rightClaw;
// don't worry about these IMUs and encoders, I will cover them later.
private BNO055IMU imu;
private boolean runThisWithEncoder = true;
// Other variable names
HardwareMap hwMap;
private ElapsedTime period = new ElapsedTime();
public Hardware2() {
hwMap = null;
this.runThisWithEncoder = true;
}
public Hardware2(boolean runThisWithEncoder) {
hwMap = null;
this.runThisWithEncoder = runThisWithEncoder;
}
public void initTeleOpIMU(HardwareMap hwMap) {
// The hardware map contains every single object defined in the rev control hub, when you go to create a config in the control hub, you set a name for the object, use that name in place of these current "hwMap.dcMotor.get("put your conf name here")"
this.hwMap = hwMap;
period.reset();
frontLeftMotor = hwMap.dcMotor.get("front_left");
frontRightMotor = hwMap.dcMotor.get("front_right");
backLeftMotor = hwMap.dcMotor.get("back_left");
backRightMotor = hwMap.dcMotor.get("back_right");
verticalLiftMotor = hwMap.dcMotor.get("vertical_lift");
leftClaw = hwMap.servo.get("leftClaw");
rightClaw = hwMap.servo.get("rightClaw");
// Initialize Motors Directions
frontLeftMotor.setDirection(DcMotor.Direction.REVERSE);
frontRightMotor.setDirection(DcMotor.Direction.FORWARD);
backLeftMotor.setDirection(DcMotor.Direction.REVERSE);
backRightMotor.setDirection(DcMotor.Direction.FORWARD);
verticalLiftMotor.setDirection(DcMotor.Direction.FORWARD);
// May use RUN_USING_ENCODER if encoders are installed (DO NOT WORRY ABOUT THIS PART)
if (runThisWithEncoder)
{
// Do if encoders are installed and you want to use them
startEncoderMode();
}
else
{
stopEncoderMode();
}
frontLeftMotor.setZeroPowerBehavior(DcMotor.ZeroPowerBehavior.BRAKE);
frontRightMotor.setZeroPowerBehavior(DcMotor.ZeroPowerBehavior.BRAKE);
backLeftMotor.setZeroPowerBehavior(DcMotor.ZeroPowerBehavior.BRAKE);
backRightMotor.setZeroPowerBehavior(DcMotor.ZeroPowerBehavior.BRAKE);
verticalLiftMotor.setZeroPowerBehavior(DcMotor.ZeroPowerBehavior.BRAKE);
// The motor power has a range of -1.0 to 1.0
// Negative is backwards, Positive is forwards, 0 is off
frontLeftMotor.setPower(0);
frontRightMotor.setPower(0);
backLeftMotor.setPower(0);
backRightMotor.setPower(0);
imu = hwMap.get(BNO055IMU.class, "imu");
BNO055IMU.Parameters parameters = new BNO055IMU.Parameters();
parameters.mode = BNO055IMU.SensorMode.IMU;
parameters.angleUnit = BNO055IMU.AngleUnit.DEGREES; //unit for turning
parameters.accelUnit = BNO055IMU.AccelUnit.METERS_PERSEC_PERSEC;
parameters.loggingEnabled = true;
imu.initialize(parameters);
}
public void startEncoderMode()
{
frontLeftMotor.setMode(DcMotor.RunMode.RUN_USING_ENCODER);
frontRightMotor.setMode(DcMotor.RunMode.RUN_USING_ENCODER);
backLeftMotor.setMode(DcMotor.RunMode.RUN_USING_ENCODER);
backRightMotor.setMode(DcMotor.RunMode.RUN_USING_ENCODER);
verticalLiftMotor.setMode(DcMotor.RunMode.RUN_USING_ENCODER);
// get the encoders reset
frontLeftMotor.setMode(DcMotor.RunMode.STOP_AND_RESET_ENCODER);
frontRightMotor.setMode(DcMotor.RunMode.STOP_AND_RESET_ENCODER);
backLeftMotor.setMode(DcMotor.RunMode.STOP_AND_RESET_ENCODER);
backRightMotor.setMode(DcMotor.RunMode.STOP_AND_RESET_ENCODER);
verticalLiftMotor.setMode(DcMotor.RunMode.STOP_AND_RESET_ENCODER);
}
public void stopEncoderMode()
{
// get the encoders reset if it was in that mode previously
frontLeftMotor.setMode(DcMotor.RunMode.STOP_AND_RESET_ENCODER);
frontRightMotor.setMode(DcMotor.RunMode.STOP_AND_RESET_ENCODER);
backLeftMotor.setMode(DcMotor.RunMode.STOP_AND_RESET_ENCODER);
backRightMotor.setMode(DcMotor.RunMode.STOP_AND_RESET_ENCODER);
verticalLiftMotor.setMode(DcMotor.RunMode.STOP_AND_RESET_ENCODER);
frontLeftMotor.setMode(DcMotor.RunMode.RUN_WITHOUT_ENCODER);
frontRightMotor.setMode(DcMotor.RunMode.RUN_WITHOUT_ENCODER);
backLeftMotor.setMode(DcMotor.RunMode.RUN_WITHOUT_ENCODER);
backRightMotor.setMode(DcMotor.RunMode.RUN_WITHOUT_ENCODER);
verticalLiftMotor.setMode(DcMotor.RunMode.RUN_USING_ENCODER);
}
//Different methods that you can use on the motors
public void setPowerOfAllMotorsTo(double power)
{
frontLeftMotor.setPower(power);
frontRightMotor.setPower(power);
backLeftMotor.setPower(power);
backRightMotor.setPower(power);
}
//Methods known as getters, these are used as a proxy to access the variables without outsiders having direct access to the variables
public double getTime(){
return period.time();
}
public DcMotor getBackLeftDrive() {
return backLeftMotor;
}
public DcMotor getLeftDrive() {
return frontLeftMotor;
}
public DcMotor getBackRightDrive() {
return backRightMotor;
}
public DcMotor getRightDrive() {
return frontRightMotor;
}
public Servo getLeftClaw() {
return leftClaw;
}
public Servo getRightClaw() {
return rightClaw;
}
public DcMotor getArm() { return verticalLiftMotor; }
public BNO055IMU getImu() {return imu;}
}